Sources MySQL
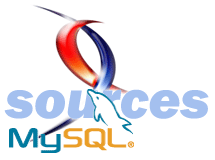
Sources MySQLConsultez toutes les sources
Nombre d'auteurs : 10, nombre de sources : 21, dernière mise à jour : 9 octobre 2010

Rappel des règles de comptages
I = 1
V = 5
X = 10
L = 50
C = 100
D = 500
M = 1000
Lorsque les symboles sont semblables on les additionne : CC = 200
Lorsque le symbole de gauche est plus petit que celui de droite on effectue une soustraction : IX = 9
Il n'est pas autorisé d'avoir plus de trois symboles semblables à la suite : IIII n'existe pas.
Compte tenu de ces règles, on ne peut compter seulement que jusqu'à MMMCMXCIX soit 3999.
Cette fonction reçoit en paramètre un chiffre arabe et retourne le chiffre romain correspondant. Si le paramètre n'est pas encodable (non compris entre 0 et 4000) elle retourne le marqueur NULL.
DROP
FUNCTION
IF
EXISTS
ArabicToRoman;
DELIMITER
$$
CREATE
FUNCTION
ArabicToRoman (
number
INT
)
RETURNS
TEXT
DETERMINISTIC
BEGIN
DECLARE
basic_roman TEXT
DEFAULT
'M,CM,D,CD,C,XC,L,XL,X,IX,V,IV,I'
;
DECLARE
basic_value TEXT
DEFAULT
'1000,900,500,400,100,90,50,40,10,9,5,4,1'
;
DECLARE
roman_string TEXT
DEFAULT
''
;
DECLARE
i INT
DEFAULT
1
;
DECLARE
roman_symbol TEXT
;
DECLARE
roman_value INT
;
SET
roman_string =
IF
(
number
<=
0
OR
number
>=
4000
, NULL
, roman_string)
;
WHILE
number
>
0
AND
number
<
4000
DO
SET
roman_symbol =
SUBSTRING_INDEX
(
SUBSTRING_INDEX
(
basic_roman,','
,i)
,','
,-
1
)
;
SET
roman_value =
SUBSTRING_INDEX
(
SUBSTRING_INDEX
(
basic_value,','
,i)
,','
,-
1
)
;
IF
number
>=
roman_value THEN
SET
roman_string =
CONCAT
(
roman_string,roman_symbol)
;
SET
number
=
number
-
roman_value;
ELSE
SET
i =
i +
1
;
END
IF
;
END
WHILE
;
RETURN
roman_string;
END
$$
DELIMITER
;
SELECT
ArabicToRoman(
'2451'
)
;
MMCDLI
Cette fonction reçoit en paramètre un chiffre romain et retourne le chiffre arabe correspondant.
DROP
FUNCTION
IF
EXISTS
RomanToArabic;
DELIMITER
$$
CREATE
FUNCTION
RomanToArabic (
roman_number TEXT
)
RETURNS
INT
DETERMINISTIC
BEGIN
DECLARE
basic_roman TEXT
DEFAULT
'M,CM,D,CD,C,XC,L,XL,X,IX,V,IV,I'
;
DECLARE
basic_value TEXT
DEFAULT
'1000,900,500,400,100,90,50,40,10,9,5,4,1'
;
DECLARE
i INT
DEFAULT
1
;
DECLARE
j INT
;
DECLARE
tmp_value INT
;
DECLARE
tmp_symbol TEXT
;
DECLARE
current_symbol TEXT
;
DECLARE
roman_length INT
DEFAULT
LENGTH
(
roman_number)
;
DECLARE
roman_symbol TEXT
;
DECLARE
roman_value INT
DEFAULT
0
;
WHILE
i <=
roman_length DO
SET
tmp_symbol =
SUBSTR
(
roman_number, i, 2
)
;
IF
(
tmp_symbol =
'CM'
OR
tmp_symbol =
'CD'
OR
tmp_symbol =
'XC'
OR
tmp_symbol =
'XL'
OR
tmp_symbol =
'IX'
OR
tmp_symbol =
'IV'
)
THEN
SET
current_symbol =
tmp_symbol;
SET
i =
i +
1
;
ELSE
SET
current_symbol =
SUBSTR
(
roman_number, i, 1
)
;
END
IF
;
SET
j =
1
;
WHILE
j <=
13
DO
SET
roman_symbol =
SUBSTRING_INDEX
(
SUBSTRING_INDEX
(
basic_roman,','
,j)
,','
,-
1
)
;
IF
roman_symbol =
current_symbol THEN
SET
tmp_value =
SUBSTRING_INDEX
(
SUBSTRING_INDEX
(
basic_value,','
,j)
,','
,-
1
)
;
SET
j =
13
;
END
IF
;
SET
j =
j +
1
;
END
WHILE
;
SET
roman_value =
roman_value +
tmp_value;
SET
i =
i +
1
;
END
WHILE
;
RETURN
roman_value;
END
$$
DELIMITER
;
SELECT
RomanToArabic(
'MMCDLI'
)
;
2451
Le but de cette fonction est de convertir tous les caractères accentués vers leurs équivalents (non accentués) et de remplacer les espaces par des tirets.
# Modification du caractère de fin d'instruction
DELIMITER
|
# On fait passer le jeux de caractères sur utf8 sinon CHAR_LENGTH('â') = 2 au lieu de 1
SET
NAMES
utf8;
# Création de la function translate
CREATE
FUNCTION
translate
(
V_string VARCHAR
(
255
)
, V_from VARCHAR
(
255
)
, V_to VARCHAR
(
255
))
RETURNS
VARCHAR
(
255
)
DETERMINISTIC
BEGIN
DECLARE
i INT
;
SET
i =
CHAR_LENGTH
(
V_from)
;
WHILE
i >
0
DO
SET
V_string =
REPLACE
(
V_string, SUBSTR
(
V_from, i, 1
)
, SUBSTR
(
V_to, i, 1
))
;
SET
i =
i -
1
;
END
WHILE
;
RETURN
V_string;
END
;
|
# Très important, Remise du delimiter standard
DELIMITER
;
SELECT
translate
(
'très accentués'
, 'éèê'
, 'eee'
)
;
+---------------------------------------------+
| translate('très accentués', 'éèê', 'eee') |
+---------------------------------------------+
| tres accentues |
+---------------------------------------------+
SELECT
translate
(
'Une très bonne initiative et à bientôt'
, 'ÁÀÂÄÃÅÇÉÈÊËÍÏÎÌÑÓÒÔÖÕÚÙÛÜÝáàâäãåçéèêëíìîïñóòôöõúùûüýÿ'
, 'AAAAAACEEEEIIIINOOOOOUUUUYaaaaaaceeeeiiiinooooouuuuyy'
)
;
+------------------------------------------------------------+
| translate('Une très bonne initiative et à bientôt', ...... |
-------------------------------------------------------------+
| Une tres bonne initiative et a bientot |
+------------------------------------------------------------+