Sources MySQL
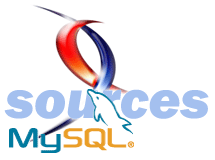
Sources MySQLConsultez toutes les sources
Nombre d'auteurs : 10, nombre de sources : 21, dernière mise à jour : 9 octobre 2010

Voici une fonction utilisateur équivalente à la fonction url_encode de PHP :
DELIMITER
$$
DROP
FUNCTION
IF
EXISTS
url_encode;$$
CREATE
FUNCTION
url_encode(
original_text TEXT
)
RETURNS
TEXT
BEGIN
DECLARE
new_text TEXT
DEFAULT
NULL
;
DECLARE
current_char VARCHAR
(
10
)
DEFAULT
''
;
DECLARE
pointer INT
DEFAULT
1
;
IF
(
original_text IS
NOT
NULL
)
THEN
SET
new_text =
''
;
WHILE
(
pointer <
LENGTH
(
original_text))
DO
SET
current_char =
MID
(
original_text, pointer, 1
)
;
IF
(
current_char =
' '
)
THEN
SET
current_char =
'+'
;
ELSEIF
(
NOT
(
ASCII
(
current_char)
BETWEEN
48
AND
57
||
ASCII
(
current_char)
BETWEEN
65
AND
90
||
ASCII
(
current_char)
BETWEEN
97
AND
122
)
)
THEN
SET
current_char =
concat
(
"%"
,lpad
(
conv
(
ascii
(
current_char)
,10
,16
)
,2
,0
))
;
END
IF
;
SET
new_text =
CONCAT
(
new_text, current_char)
;
SET
pointer =
pointer +
1
;
END
WHILE
;
END
IF
;
RETURN
new_text;
END
;$$
DELIMITER
;
SELECT
url_encode(
'http://www.google.fr/search?source=ig&q=du+texte'
)
;
http%3A%2F%2Fwww.google.fr%2Fsearch%3Fsource%3Dig%26q%3Ddu%2Btexte
Voici une fonction utilisateur équivalente à la fonction url_decode de PHP :
DELIMITER
$$
DROP
FUNCTION
IF
EXISTS
url_decode;$$
CREATE
FUNCTION
url_decode(
original_text TEXT
)
RETURNS
TEXT
BEGIN
DECLARE
new_text text
default
null
;
DECLARE
pointer int
default
1
;
SET
new_text =
REPLACE
(
original_text, '+'
, ' '
)
;
WHILE
(
LOCATE
(
"%"
,new_text,pointer)
<>
0
)
&&
(
pointer <
length
(
new_text))
DO
SET
pointer =
LOCATE
(
"%"
,new_text,pointer)
;
SET
new_text =
CONCAT
(
LEFT
(
new_text, pointer -
1
)
, CHAR
(
CONV
(
MID
(
new_text, pointer +
1
, 2
)
, 16
, 10
))
, RIGHT
(
new_text, LENGHT(
new_text)
-
(
pointer+
2
)))
;
SET
pointer =
pointer +
1
;
END
WHILE
;
RETURN
new_text;
END
;$$
DELIMITER
;
SELECT
url_encode(
'http%3A%2F%2Fwww.google.fr%2Fsearch%3Fsource%3Dig%26q%3Ddu%2Btexte'
)
;
http://www.google.fr/search?source=ig&q=du+texte
Voici une fonction utilisateur équivalente à la fonction url_decode de PHP :
DELIMITER
$$
DROP
FUNCTION
IF
EXISTS
url_decode;$$
CREATE
FUNCTION
url_decode(
p_text TEXT
)
RETURNS
TEXT
BEGIN
DECLARE
i INT
DEFAULT
1
;
DECLARE
new_texte TEXT
DEFAULT
p_text;
DECLARE
texte_deb TEXT
;
DECLARE
texte_fin TEXT
;
DECLARE
convertion TEXT
;
DECLARE
sub TEXT
;
REPEAT
SET
sub =
SUBSTRING
(
new_texte, i, 3
)
;
IF
(
LENGTH
(
sub )
>
2
)
THEN
IF
(
sub REGEXP
'^%[A-Fa-f0-9]{2}$'
)
THEN
SET
convertion =
CHAR
(
CONV
(
SUBSTRING
(
sub, 2
)
, 16
, 10
)
)
;
SET
texte_deb =
SUBSTRING
(
new_texte, 1
, i -
1
)
;
SET
texte_fin =
SUBSTRING
(
new_texte, i +
3
)
;
SET
new_texte =
CONCAT
(
texte_deb, convertion, texte_fin )
;
END
IF
;
END
IF
;
SET
i =
i +
1
;
UNTIL
(
LENGTH
(
sub )
<=
2
)
END
REPEAT
;
RETURN
new_texte;
END
;$$
DELIMITER
;
SELECT
url_encode(
'http%3A%2F%2Fwww.google.fr%2Fsearch%3Fsource%3Dig%26q%3Ddu%2Btexte'
)
;
http://www.google.fr/search?source=ig&q=du+texte
Voici une fonction utilisateur équivalente à la fonction REPLACE mais insensible à la casse :
DELIMITER
$$
DROP
FUNCTION
IF
EXISTS
replace_ci;$$
CREATE
FUNCTION
replace_ci(
str TEXT
, needle CHAR
(
255
)
, str_rep CHAR
(
255
))
RETURNS
TEXT
DETERMINISTIC
BEGIN
DECLARE
return_str TEXT
DEFAULT
''
;
DECLARE
lower_str TEXT
;
DECLARE
lower_needle TEXT
;
DECLARE
pos INT
DEFAULT
1
;
DECLARE
old_pos INT
DEFAULT
1
;
SELECT
LOWER
(
str)
INTO
lower_str;
SELECT
LOWER
(
needle)
INTO
lower_needle;
SELECT
LOCATE
(
lower_needle, lower_str, pos)
INTO
pos;
WHILE
(
pos >
0
)
DO
SELECT
CONCAT
(
return_str, substr
(
str, old_pos, pos-
old_pos)
, str_rep)
INTO
return_str;
SELECT
pos +
CHAR_LENGHT(
needle)
INTO
pos;
SELECT
pos INTO
old_pos;
SELECT
LOCATE
(
lower_needle, lower_str, pos)
INTO
pos;
END
WHILE
;
SELECT
CONCAT
(
return_str, SUBSTR
(
str, old_pos, char_length
(
str)))
INTO
return_str;
RETURN
return_str;
END
$$
DELIMITER
;
SELECT
replace_ci(
'mySQL'
, 'M'
, 'M'
)
;
MySQL